Thank you! Your submission has been received!
Oops! Something went wrong while submitting the form.
Stream
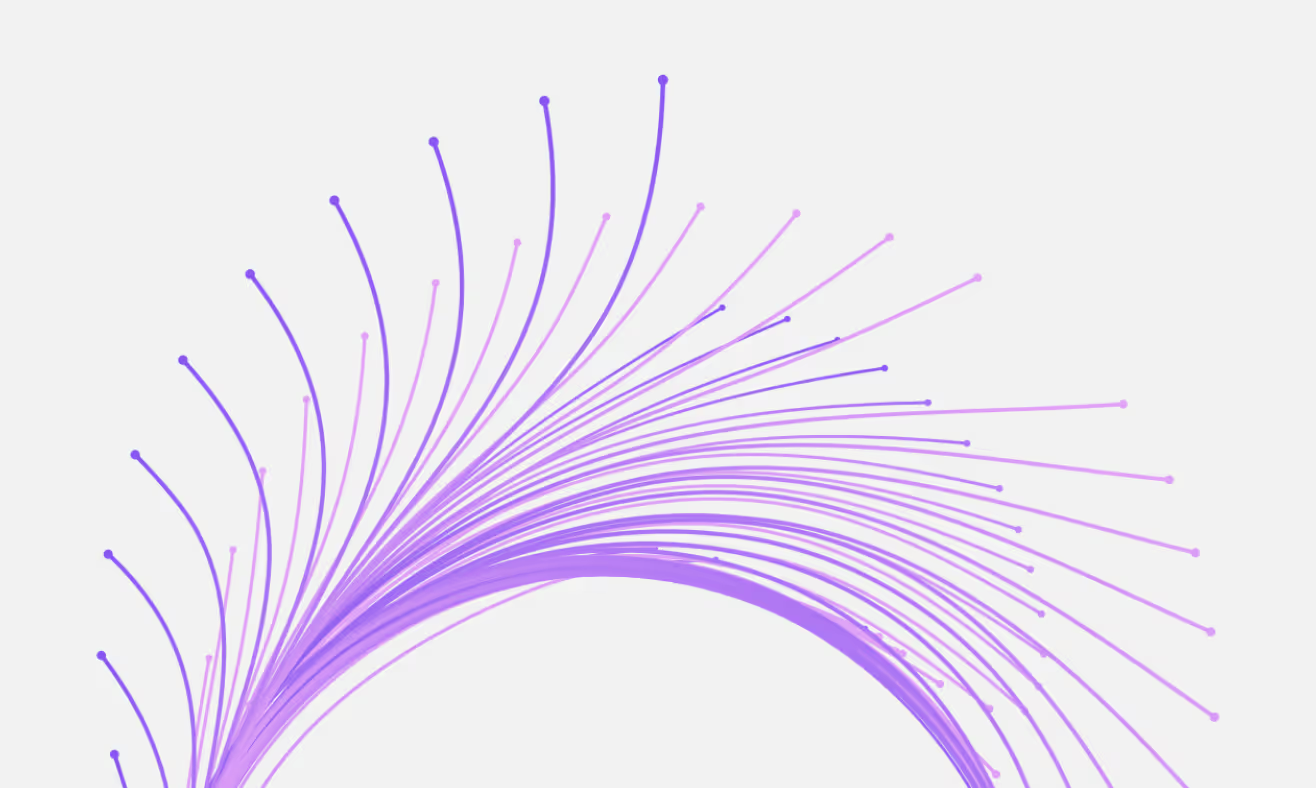
Stream
Stream is a data-driven communication platform that pushes the boundaries of global trade excellence by empowering teams and driving profitability. It lightens the manual work of managing email, connects to your other systems and presents data so teams can make better, faster decisions.
Pulse

Pulse
Pulse sits on top of the email inbox, transforming its data into a single, consolidated and customisable view. It allows charterers to find deals faster than the competition and fix with confidence. By unlocking critical data, Pulse turns challenges into opportunities.